A Deep Dive into C Programming: Call by Value, Call by Reference, and Manipulating C Strings
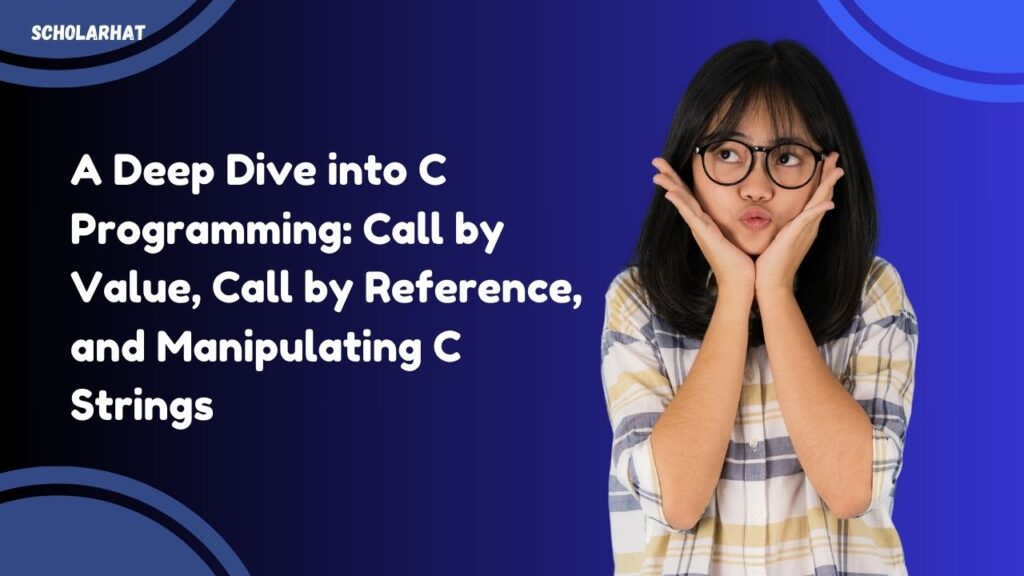
C programming remains a fundamental skill for developers, offering low-level control over system resources and memory. Key concepts such as call by value, call by reference, and string manipulation in C are crucial for efficient coding and performance optimization. This comprehensive guide explores these essential aspects of C programming, enhancing both your understanding and proficiency.
Call by Value and Call by Reference in C
Understanding Call by Value
In the call by value method, the function receives the values of arguments in separate memory locations. Any modifications made within the function do not affect the actual parameters outside of the function. This method is preferred for small and simple data types where the overhead of copying data is negligible.
Example of Call by Value:
#include <stdio.h>
void addTen(int x) {
x += 10; // changes affect only the local copy
}
int main() {
int num = 5;
addTen(num);
printf(“%d”, num); // Output will be 5
return 0;
}
This example demonstrates that changes inside the addTen function do not impact the original variable num.
Understanding Call by Reference
Call by reference, in contrast, allows a function to modify the actual parameters by passing the addresses of variables. This is done using pointers in C. This method is more efficient for large data structures like arrays, where copying large amounts of data would be computationally expensive.
Example of Call by Reference:
#include <stdio.h>
void addTen(int *x) {
*x += 10; // changes affect the actual variable
}
int main() {
int num = 5;
addTen(&num);
printf(“%d”, num); // Output will be 15
return 0;
}
In this example, the actual value of num is modified through its pointer, demonstrating how call by reference works.
For a deeper understanding of these concepts, visit the detailed guide on call by value and call by reference in C.
Manipulating C Strings
C does not natively support strings as a data type. Instead, strings are implemented as arrays of characters terminated by a null character (). Manipulating strings in C can be tricky due to this representation.
String Initialization
Strings in C are typically declared as arrays of characters:
char str[6] = “hello”; // ‘h’, ‘e’, ‘l’, ‘l’, ‘o’, ”
Common String Operations
C’s standard library provides several functions to manipulate strings, which include copying, concatenation, comparison, and calculation of length. Here’s how some of these operations are typically handled:
String Copy:
char src[50], dest[50];
strcpy(src, “This is source”);
strcpy(dest, src);
String Concatenation:
char dest[50] = “Hello “;
strcat(dest, “World”); // dest now contains “Hello World”
String Length:
char str[50] = “Hello World”;
int length = strlen(str); // length will be 11
For a comprehensive understanding of string operations in C, including more advanced topics, you can refer to a detailed discussion on C string manipulation.
Conclusion
Mastering call by value, call by reference, and string manipulation in C will significantly enhance your capability to write efficient and effective C programs. These foundational concepts are critical for tackling more complex programming challenges in C and are indispensable for anyone looking to deepen their understanding of system-level programming.