React Form Validation: Best Practices and Techniques
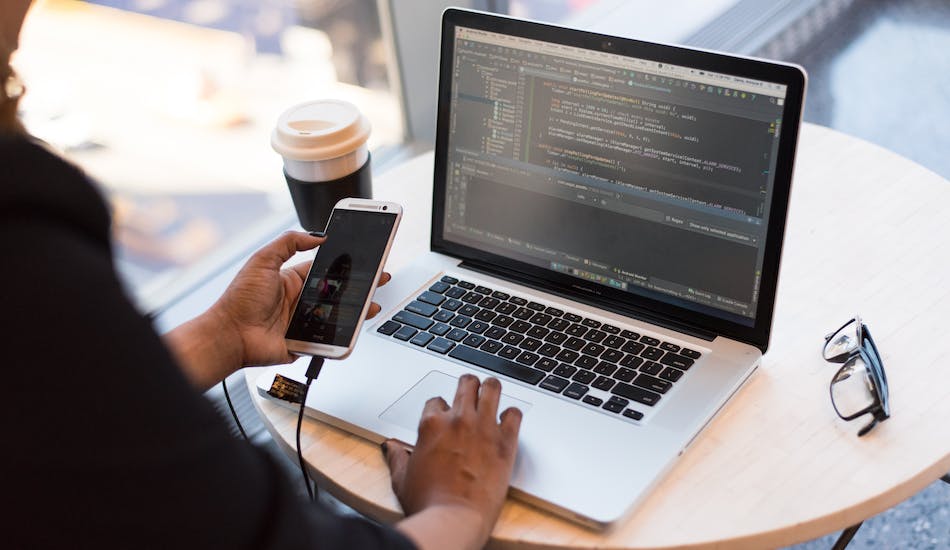
Form validation certainly matters as a part of modern web development. It ensures that not just the provided data, but also the data adopted by the users follows specific predefined criteria and rules before the data processing or storage takes place. React (among the most popular front-end libraries) makes it possible for developers to create forms that are both visually attractive and user-friendly.
Defining Form Validation
Form validation can be broadly categorized into two types: client-side and server-side validation. Client-side validation happens within the browser before the data is sent to the server. It enables immediate feedback to users and is less demanding on servers. Server-side validation is performed by the server, ensuring that the data conforms to the required criteria before it’s processed or stored.
For developers who want to make their apps effective and more responsive, it is necessary to get the client-side validation in React right. It’s also an important skill for those looking to hire React engineer since it significantly improves the user experience by supplying immediate and contextual feedback.
Simple Techniques for Effective Validation
Use State to Manage Form Data
React’s state management is a powerful tool for handling form inputs. By maintaining state from the form values, it is possible to build in validation logic that immediately reacts to changes in state. With this method, there will be instant validation responses as the participant interacts with the form.
Leverage Conditional Rendering
Conditional rendering in React can be used to show or hide validation messages based on the form’s state. This technique allows you to create a more interactive and responsive user experience, as feedback is given instantaneously based on the user’s inputs.
Take Advantage of React’s Built-in Events
React’s built-in form events, such as onChange, onSubmit, and onBlur, can be utilized to trigger validation logic. For instance, using onBlur can help in validating individual fields when the user moves away from an input field, ensuring that errors are caught early in the form-filling process.
Validation Logic
When it comes to implementing validation logic, there are a couple of approaches you can take:
Manual Validation
Manual validation involves writing custom validation functions for your forms. This method allows you to validate the input by all means which makes it possible to rigidly define rules for each field. Although it requires more code, manual validation is highly flexible and you can tailor it to meet any form requirement.
Validation Library
Several React-specific validation libraries, such as Formik, Yup, and React Hook Form, can simplify the validation process. These libraries allow you to use a library of functions and tools for form validation which usually reduces the amount of code that you need to write yourself. It has schema-based validations that you can use to make your app more readable and cleaner.
Common Validation Techniques
Regardless of the method you choose, certain validation techniques are commonly used in React forms:
-
Required Fields
Ensure that the user fills out all necessary fields.
-
Pattern Matching
Use regular expressions to validate the format of the input (e.g., email addresses, and phone numbers).
-
Length Validation
Check the length of the input to ensure it meets certain criteria (e.g., password strength).
-
Custom Validators
Add your own functions to validate more complex logic, like checking whether the username you are posting is valid or not.
Best Practices for User-Friendly Validation
To create a positive user experience, consider the following best practices:
-
Immediate Feedback
Provide real-time validation feedback to guide users as they fill out the form.
-
Clear Error Messages
Use clear and concise messages to communicate validation errors.
-
Accessibility
Ensure that your validation feedback is accessible to all users, including those using screen readers.
Conclusion
Form validation in React brings about interactive and user-friendly web applications. A validation form that is thoroughly verified doesn’t just improve the user experience but also increases the reliability of the gathered data. Keep your validation logic simple, user-friendly, and, most importantly, aligned with the needs of your users.